C++ OpenCV zeroPaddingCrop
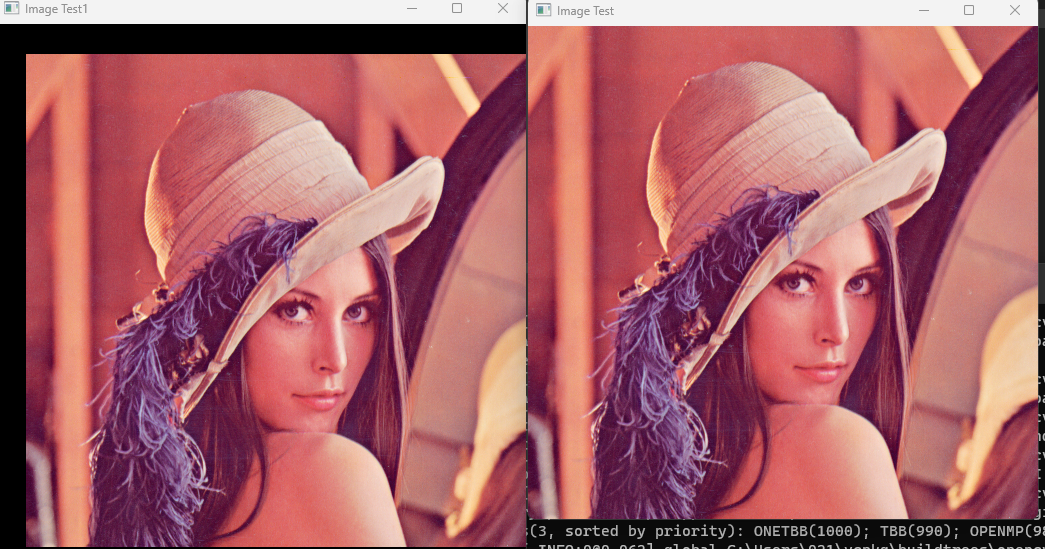
#include <iostream>
#include <fstream>
#include <iostream>
#include <algorithm>
#include <opencv2/opencv.hpp>
using namespace std;
cv::Mat ZeroPaddingCrop(cv::Mat image, int x1, int y1, int x2, int y2) {
auto imageRect = cv::Rect({}, image.size());
auto roi = cv::Rect(x1, y1, x2 - x1, y2 - y1);
auto intersection = imageRect & roi;
cv::Rect interRoi = intersection - roi.tl();
cv::Mat crop = cv::Mat::zeros(roi.size(), image.type());
image(intersection).copyTo(crop(interRoi));
return crop;
}
int main(int argc, char** argv) {
cv::Mat image = cv::imread("1.png");
auto image1 = ZeroPaddingCrop(image, -30, -30, 500, 500);
imshow("Image Test", image);
imshow("Image Test1", image1);
cv::waitKey(0);
return 0;
}
Crop할때 원본 이미지를 좌표를 넘기는 경우가 있는데 이때 사용하는 전략중에 하나가 ZeroPaddingCrop이다.
자주 사용하다보니 남긴다.